Developers
Whether you're a startup or a global enterprise,
learn how to integrate with Fern Software to intelligently power your integrations.
learn how to integrate with Fern Software to intelligently power your integrations.
Getting started
Our software opens up possibilities for your organization like never before. Our APIs and integrations can be used from many technologies, here are just a few examples of how to begin.
Start Building{
client.DefaultRequestHeaders.Add("X-Fern-Token", API_TOKEN_VALUE);
client.DefaultRequestHeaders.Add("User-Agent", "NameOfYourApplication");
var response =
await client.GetAsync("https://api.fernsoftware.com/applications/");
var result = JsonConvert.DeserializeObject<Application[]>(
await response.Content.ReadAsStringAsync());
foreach (var entity in result)
{
Console.WriteLine(entity.Id);
}
}
xhr.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
const entities = JSON.parse(xhr.responseText);
for (let i = 0; i < entities.length; i++) {
console.log(entities[i].Id);
}
}
};
xhr.setRequestHeader("X-Fern-Token", API_TOKEN_VALUE);
xhr.setRequestHeader("User-Agent", "NameOfYourApplication");
xhr.open("GET", "https://api.fernsoftware.com/applications/", true);
xhr.send();
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("X-Fern-Token", API_TOKEN_VALUE);
connection.setRequestProperty("User-Agent", "NameOfYourApplication");
String line;
BufferedReader reader = new BufferedRead(new InputStreamReader(connection.getInputStream()));
StringBuffer response = new StringBuffer();
while ((line = reader.readLine()) != null) {
response.append(line);
}
input.close();
JSONArray entities = new JSONArray(response.toString());
entities.forEach(item -> {
System.out.println(item.getString("Id"));
});
Partners and integrations
Our software is tightly integrated with third-parties so you get the solution you need.
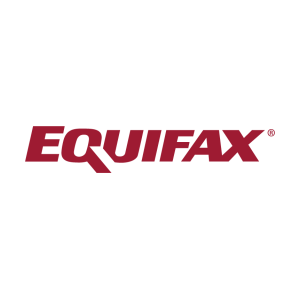
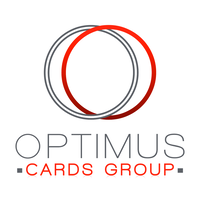
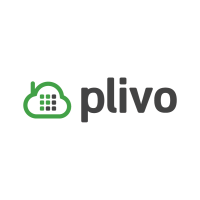
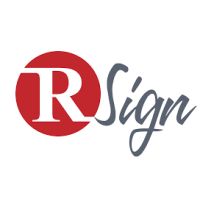
API Documentation
Our APIs are designed around REST. This offers a predictable resource-oriented URL scheme and produces JSON encoded responses using standard HTTP status codes, authentication and verbs.
DocumentationPlatform Status
We deliver mission critical data around the clock. Learn about changes and disruptions to our API including planned outages and maintenance windows to stay in the know.
Check StatusBuild with Fern Software
We're reinventing how financial software can be developed. Work with us on GitHub on open source projects or use some of the tools we use ourselves to create custom solutions.
Visit GitHub